Introduction to the XOR Operator
Table of Contents
Hey there, coding enthusiast! Ever heard of the XOR operator? If not, you’re in for a treat! XOR, which stands for “exclusive OR,” is one of those cool tools in the programmer’s toolkit that’s both fascinating and super useful. Whether you’re diving into the world of binary operations or just looking for a neat trick to impress your coding buddies, XOR has got your back.
So, what’s the magic behind XOR? Well, XOR is a type of logical operator that gives us a True
output when either of its inputs is True
, but not when both are. Sounds a bit puzzling? Don’t worry; let’s break it down with a truth table:
Input A | Input B | A XOR B |
---|---|---|
True | True | False |
True | False | True |
False | True | True |
False | False | False |
Look at that table! If both inputs (A and B) are True
, XOR says, “Nah, I’m gonna be False
.” But if only one of them is True
, XOR is all like, “Yup, that’s a True
for me!”
In the world of 1s and 0s (binary), XOR plays a similar game. It’s like a fun puzzle where it checks two bits and lights up when they’re different.
Stay with me, and by the end of this article, you’ll be an XOR wizard, casting spells in your code and making magic happen!
Ready to dive deeper? Let’s go!
Python XOR Operator
Alright, moving on to the next exciting chapter of our XOR journey!
What is the XOR Operator?
In simple terms, XOR is like that friend who can’t decide between two ice cream flavors and ends up choosing a totally different one! It looks at two inputs and says, “If you both are different, I’m on board. But if you’re the same? Nope, count me out!”
Mathematical Notation vs. Python Notation
Now, here’s where things get a tad bit technical, but stick with me! In the world of mathematics, XOR is represented by this fancy symbol ‘⊕’. But Python, being the cool language it is, decided to keep things simple and went with ‘^’. So, every time you see ‘^’ in Python, just remember it’s doing the XOR magic!
Table 1: Comparison of XOR notations in Mathematics and Python
Notation Type | Symbol |
---|---|
Mathematics | ⊕ |
Python | ^ |
And now, for the grand reveal…
Figure 1: Visual representation of XOR operation
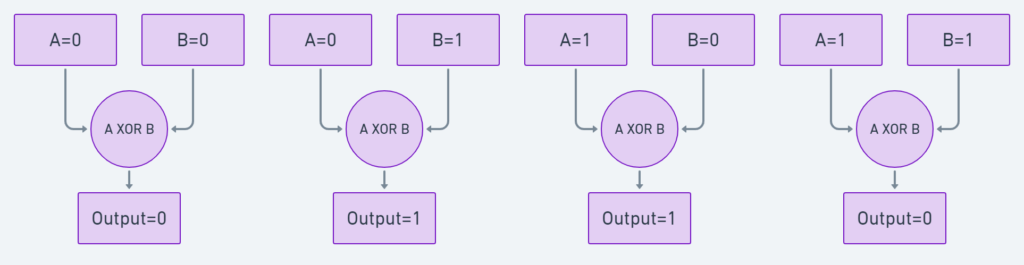
Look at that beauty! The diagram shows how XOR checks the inputs. When the inputs are different (like 0 and 1 or 1 and 0), XOR gives a thumbs up (or a 1 in binary terms). But when the inputs are the same (both 0s or both 1s), XOR is like, “Nah, I’m good,” and gives a 0.
Feeling like an XOR pro yet? We’re just getting started! Onward to more XOR adventures!
XOR of Integers
Buckle up, math enthusiasts! We’re diving deep into the world of integers and how XOR plays with them.
Bitwise Operations: A Quick Intro
Before we jump into XOR, let’s take a brief detour into the realm of bitwise operations. Imagine numbers as strings of 1s and 0s (binary form). Bitwise operations, as the name suggests, operate on these individual bits. It’s like giving each bit a tiny task to perform!
How XOR Compares Bits of Two Integers
When XOR looks at two integers, it breaks them down into their binary form. Then, it goes bit by bit, comparing each pair. If the bits are different, XOR gives a 1. If they’re the same, it gives a 0. Simple, right?
Let’s Dive into an Example!
Consider the integers 15 and 32. In binary, 15 is 00001111
and 32 is 00100000
. Now, let’s see what happens when XOR dances with them:
15: 00001111
32: 00100000
Result: 00101111 (which is 47 in decimal!)
So, 15 ^ 32 = 47
Figure 2: Bitwise representation of 15 and 32 and their XOR result
Common XOR Operations with Integers
To wrap up this section, here’s a quick list of some common XOR operations and their results:
- 5 ^ 3 = 6: In binary, it’s
0101 ^ 0011 = 0110
. - 7 ^ 8 = 15: In binary, it’s
0111 ^ 1000 = 1111
. - 10 ^ 2 = 8: In binary, it’s
1010 ^ 0010 = 1000
.
And there you have it! The magic of XOR with integers. Ready for more XOR fun? Let’s keep the momentum going!
XOR of Booleans
Welcome to the world of Booleans, where everything is either True
or False
!
In this section, we’ll explore how XOR interacts with these binary buddies.
How XOR Works with Boolean Values
Booleans are like the light switches of the programming world: they’re either ON (True
) or OFF (False
). XOR, being the curious operator it is, checks these switches and lights up only when they’re in opposite states. If both switches are the same, XOR stays dark.
Let’s Break It Down with a Truth Table!
Table 2: Truth table for XOR operation with booleans
Input A | Input B | A XOR B |
---|---|---|
True | True | False |
True | False | True |
False | True | True |
False | False | False |
As you can see, XOR only shines bright (returns True
) when the inputs are different. If they’re the same, it’s lights out (returns False
).
A Quick Example to Seal the Deal
Let’s take a real-world example. Imagine you have two light switches. XOR is like a bulb that lights up only when one switch is ON and the other is OFF. So, if you turn on the first switch (True
) and leave the second one off (False
), the bulb will light up! In Python terms, this is:
True ^ False = True
And there you have it! The beauty of XOR with Booleans. It’s simple, elegant, and super handy in many coding scenarios. Ready to dive even deeper into the XOR universe? Let’s go!
XOR Swap Algorithm
Ever been in a situation where you wanted to swap two items, but didn’t have a third hand to help? Well, the XOR Swap Algorithm is like a magical trick that lets you swap two numbers without needing an extra variable (or hand)!
Introduction to the XOR Swap Algorithm
The XOR Swap Algorithm is a nifty technique that uses the properties of the XOR operation to swap the values of two variables. No temporary storage needed! It’s like a juggling act where you never drop the ball.
Why Use XOR for Swapping?
Traditional swapping methods require a temporary variable to hold one of the values while the swap is being performed. This means extra memory and extra steps. But with XOR, we can perform the swap directly, making the process faster and more efficient. It’s like taking a shortcut on your daily commute!
Let’s Dive into the Algorithm!
Here’s a step-by-step breakdown of the XOR Swap Algorithm:
- Step 1: Take two numbers, let’s call them
a
andb
. - Step 2: Update the value of
a
to be the XOR ofa
andb
. - Step 3: Update the value of
b
to be the XOR of the newa
andb
. - Step 4: Finally, update the value of
a
to be the XOR ofa
and the newb
.
Voilà! a
and b
have now swapped places!
Code Snippet: Demonstrating the XOR Swap Algorithm
a = 5 # Let's say a is 5 b = 10 # and b is 10 # Now, let's perform the XOR swap magic! a = a ^ b b = a ^ b a = a ^ b print(a) # This will now print 10 print(b) # This will now print 5
Figure 3: Flowchart explaining the XOR swap algorithm
And that’s the XOR Swap Algorithm in all its glory! It’s a testament to the power and versatility of the XOR operation. Ready to explore more XOR wonders? Let’s keep the adventure going!
XOR Function in Python
Hey there, Pythonistas! Ever wished for a toolbox that makes working with operations like XOR even easier? Well, Python’s got you covered with the operator
module. Let’s dive in and see what it’s all about!
Introduction to the operator
Module in Python (Python’s Exclusive OR Operator)
The operator
module in Python is like a Swiss Army knife for programmers. It provides a set of predefined functions that correspond to Python’s in-built operators. So, instead of using symbols like ^
, +
, or -
, you can use their function equivalents. Handy, right?
Using the XOR Function from the operator
Module
Within the operator
module, there’s a function called xor
that lets you perform the XOR operation without using the ^
symbol. It’s especially useful when you want your code to be more readable or when working with functions that expect callable arguments.
Code Snippet: Demonstrating the use of operator.xor(12, 13)
import operator result = operator.xor(12, 13) print(result) # This will print 1
See? It’s as simple as calling a function!
Other Useful Functions in the operator
Module
The operator
module isn’t just about XOR. It’s packed with functions for various bitwise operations. Here’s a quick list of some of them:
and_
: Bitwise AND operation.or_
: Bitwise OR operation.invert
: Bitwise inversion (NOT operation).lshift
: Left bitwise shift.rshift
: Right bitwise shift.
These functions can be super handy when you’re diving deep into the world of bitwise operations in Python. So, next time you’re tinkering with bits and bytes, remember the operator
module has got your back!
Ready to continue our XOR journey? There’s still more to explore, so let’s keep the momentum going!
Conclusion and Further Exploration
Wow, what a journey we’ve been on together! From understanding the basics of the XOR operator to diving deep into its applications in Python, we’ve covered a lot of ground. Let’s take a moment to reflect and see where we can go from here.
Key Takeaways
- XOR Basics: XOR, or exclusive OR, is a bitwise operator that returns 1 when the bits being compared are different and 0 when they’re the same.
- Python’s XOR: In Python, XOR is represented by the
^
symbol, making it easy to perform bitwise operations on integers. - Booleans and XOR: XOR can also work with boolean values, returning
True
when the values are different andFalse
when they’re the same. - Swapping with XOR: The XOR swap algorithm is a clever way to swap two numbers without needing a temporary variable.
- The
operator
Module: Python’soperator
module provides a functional way to use XOR and other bitwise operations, making your code more readable and versatile.
Further Exploration
If you’re as fascinated by XOR as we are, there’s still so much more to explore:
- Other Bitwise Operators: Dive into other bitwise operators like AND, OR, and NOT to see how they compare with XOR.
- Applications of XOR: XOR has many real-world applications, from error detection in data transmission to cryptography. Explore these areas to see XOR in action!
- Advanced Python Modules: Beyond the
operator
module, Python offers a plethora of modules and libraries for advanced operations. Dive into the Python documentation to discover more.
Wrapping Up
Thank you for joining us on this XOR adventure! We hope you found it enlightening and fun. Remember, the world of programming is vast and full of wonders. Keep exploring, keep learning, and most importantly, keep coding!
Until next time, happy coding!
If you would like to learn data visualization in python, you can check out this post on creating boxplot using Python
Frequently Asked Questions (FAQs) on XOR
Ah, the world of XOR is vast and intriguing, and it’s natural to have questions. Let’s address some of the most commonly asked questions about the XOR operator and its applications in Python.
1. Why is XOR called “exclusive OR”?
XOR stands for “exclusive OR” because it returns True
(or 1) exclusively when one of the inputs is True
(or 1) and the other is False
(or 0). It’s like an exclusive party where only opposites are allowed!
2. Can I use XOR with data types other than integers and booleans in Python?
While the primary use of XOR in Python is with integers and booleans, you can technically use it with any data type that supports bitwise operations. However, the results might not always be intuitive, so tread carefully!
3. Is the XOR swap algorithm faster than traditional swapping methods?
The XOR swap algorithm eliminates the need for a temporary variable, which can be more memory-efficient. However, modern compilers often optimize traditional swapping, making the speed difference negligible. It’s more of a cool trick than a performance booster!
4. Why would I use the operator
module instead of the ^
symbol for XOR?
Using the operator
module can make your code more readable, especially for those unfamiliar with bitwise operators. It’s also handy when working with functions that expect callable arguments. Think of it as an alternative tool in your coding toolbox!
5. Are there other bitwise operators in Python similar to XOR?
Absolutely! Python supports a range of bitwise operators, including AND (&
), OR (|
), NOT (~
), right shift (>>
), and left shift (<<
). Each has its own unique properties and applications.