Introduction
Lately I was working on a project where I had to pass some sample data to test my system.
I wondered what I can do to get such data. So, I thought to write a python program to get this work done.
Today I will be sharing with you what I did to get random phone numbers along with the country code.
So If you’re a Python enthusiast, eager to learn a new trick, or simply someone looking for an easy way to generate random phone numbers for testing purposes, you’re in the right place. Today, I’ll walk you through the same.
Table of Contents
Learning Outcome
By the end of this guide, you’ll have learned how to create a Python program that generates random phone numbers based on user input. This will include understanding how to take user input in Python, generate random numbers, and format them appropriately.
Real Life Applications
This concept can be used in numerous scenarios. If you’re a developer working on a software or an application, you can use this to create dummy data for testing. It can also be handy in machine learning and data analysis projects, where you may need a large number of phone numbers for simulations.
Let’s Get Coding
Python, with its vast library support and easy-to-understand syntax, makes it quite straightforward to generate random phone numbers. We will see two methods to do this first without any external library and then with an external library. Let’s dive in!Generate Random Phone Numbers using Python – 2 Methods in 2024
Method 1: Without Using Third-party Libraries
You don’t always need to rely on external libraries. Python’s inbuilt `random` module can be a powerful ally. Here’s how you can generate random phone numbers using Python without any additional libraries:
# First, we import the 'random' library. This library is used to generate random numbers or to make random choices. import random # This is a function to generate phone numbers. It takes in two parameters: 'country_code' and 'num_numbers'. # 'country_code' is the code you want to attach to the phone numbers, like +1 for the US, +91 for India, etc. # 'num_numbers' is the number of phone numbers you want to generate. def generate_phone_numbers(country_code, num_numbers): # We create an empty list called 'phone_numbers' to hold all the generated phone numbers. phone_numbers = [] # This is a loop that will repeat as many times as the 'num_numbers' variable. for _ in range(num_numbers): # We generate a 10-digit number. We use the 'random.choices' function to select digits (0-9) randomly, # and 'join' them together into a string. 'k=10' means we want to choose 10 items. number = "".join(random.choices("0123456789", k=10)) # We then add the country code in front of the number, and append it to our 'phone_numbers' list. phone_numbers.append(f'{country_code}{number}') # Once the loop has finished, we return the list of generated phone numbers. return phone_numbers # This is our main function where the program starts. def main(): # We ask the user to enter a country code. country_code = input("Enter the country code: ") # We ask the user to enter how many phone numbers they want to generate. # We convert this input to an integer because 'input' function returns a string by default. num_numbers = int(input("Enter how many phone numbers to generate: ")) # We call our 'generate_phone_numbers' function, passing in the user's inputs as arguments. # The returned list of phone numbers is stored in the 'phone_numbers' variable. phone_numbers = generate_phone_numbers(country_code, num_numbers) # We then loop over the 'phone_numbers' list, and print each phone number one by one. for phone_number in phone_numbers: print(phone_number) # This line checks if this script is being run directly or it's being imported into another script. # If it's being run directly, it calls the 'main' function to start the program. if __name__ == "__main__": main()
This script generates 10-digit phone numbers, each composed of randomly selected digits, and prepends the user-inputted country code to each. This is a simple and engaging way to produce random phone numbers.
You will get following results when you run it. I am using Jupyter notebook to do so, you can use any software
1) Step 1 – When you run it, the program will ask you country code, here I entered +91 which is country code of India.

2) Step 2 – Now once you press enter, you will find next input option asking you to enter number of records. Here I added 10.

3) Step 3 – Once you press enter, you will see the 10 random phone numbers being generated.
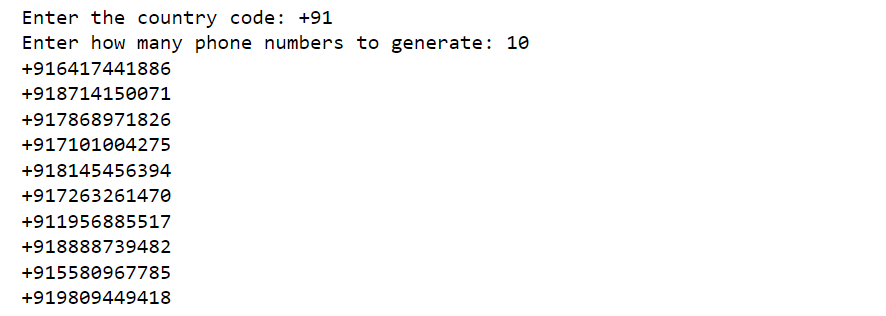
Method 2 – With Third-party Libraries
Here we will use an external library of python called `Faker`. To know more about this library you can check out its detailed documentation using the Link
So first we need to install faker library. To do so run the following command on terminal or in a cell in Jupyter notebook.
# Faker is a Python library that allows you to create fake data - in this case, we are using it to generate random numbers. pip install Faker
I did this in Jupyter notebook and got the following output

Now we will enter the actual code to generate random numbers.
# We're importing the 'random' library, which is used to generate random numbers in Python. import random # We import the 'Faker' class from the 'faker' library. from faker import Faker # This function generates random phone numbers. # It takes as parameters the 'country_code' and the number of phone numbers to generate 'num_numbers'. def generate_phone_numbers(country_code, num_numbers): # We create an instance of the 'Faker' class. This instance has methods we can use to create fake data. fake = Faker() # The 'seed' method is used to initialize the pseudorandom number generator. # This means that the generated fake data will be the same each time the program runs. Faker.seed(4321) # We create an empty list called 'phone_numbers'. This will store the phone numbers that we generate. phone_numbers = [] # This loop will run 'num_numbers' times. for _ in range(num_numbers): # We use the 'random_number' method of the 'fake' instance to generate a 10-digit number. # The 'digits' parameter specifies the number of digits in the number, # and 'fix_len=True' ensures that the number will always have exactly 10 digits. number = fake.random_number(digits=10, fix_len=True) # We add the country code to the start of the number and then add the complete phone number to our list. phone_numbers.append(f'{country_code}{number}') # After all phone numbers have been generated and added to the list, we return this list. return phone_numbers # This is the main function that is run when the program starts. def main(): # We ask the user for the country code and the number of phone numbers to generate. country_code = input("Enter the country code: ") num_numbers = int(input("Enter how many phone numbers to generate: ")) # We call the 'generate_phone_numbers' function with the user's inputs. # The function returns a list of phone numbers, which we store in the 'phone_numbers' variable. phone_numbers = generate_phone_numbers(country_code, num_numbers) # We print each phone number in the list. for phone_number in phone_numbers: print(phone_number) # This line checks if this script is the main program and not being imported by another script. # If it is the main program, it runs the 'main' function. if __name__ == "__main__": main()
You will get following results when you run it. I am using Jupyter notebook to do so, you can use any software: Generate Random Phone Numbers using Python – 2 Methods in 2024
1) Step 1 – When you run it, the program will ask you country code, here I entered +91 which is country code of India.

2) Step 2 – Now once you press enter, you will find next input option asking you to enter number of records. Here I added 10.

3) Step 3 – Once you press enter, you will see the 10 random phone numbers being generated.
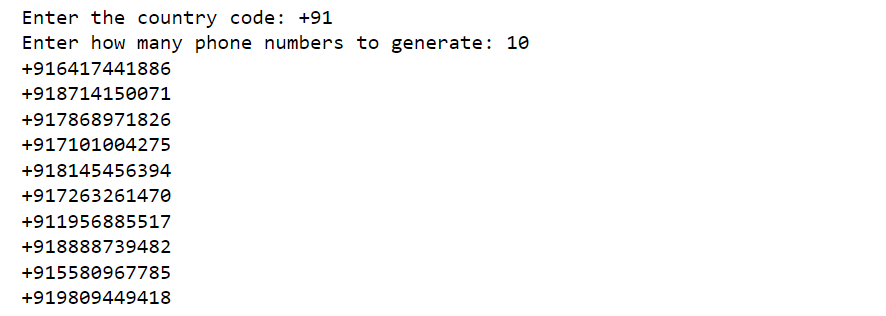
Generate Random Phone Numbers using Python – 2 Methods in 2024
Conclusion
That’s all for this tutorial. I hope you enjoyed it. Generating random phone numbers can serve many purposes and it’s a concept that can be applied to several real-life scenarios. I hope this tutorial has equipped you with an extra tool in your Python toolbox.
Python offers much more to explore beyond generating random phone numbers. If you want to delve deeper into the fascinating world of Python and programming, don’t hesitate to connect.
You can check out more of my python tutorials at https://statssy.com/category/software-tutorial/python/
Further Quests: Level Up Your Data Game Python
Here’s a treasure trove of resources that are as binge-worthy as the latest Netflix series.
Books & Articles
Statistics Fundamentals
- Forecast Like a Pro with Exponential Smoothing in Excel
- Mean vs Median: The Ultimate Showdown
- Simple Linear Regression and Residuals: A Step-by-Step Guide
- Essential Data Terminology for Business Analytics
- Different Types of Statistical Analysis Techniques
- Understanding Residuals in Statistics
- Empirical Rule Calculator in Statistics
- How to Find the Probability of A or B with Examples
- Understanding Skewed Distributions
- Levels of Measurement in Statistics
- Understanding Z-Score in Business Statistics
- What is Spearman’s Rank Correlation Coefficient
- How to Do Dsum Excel Function with And Criteria
- How to Calculate Pearson Correlation Coefficient by Hand
R Programming
- Simple Linear Regression in R: A Super Chill Guide
- Mastering the Use of Letters in R Programming
- How to Calculate Coefficient of Variation in R Language
- How to Create and Interpret Descriptive Statistics in R
- How to Create and Interpret the Boxplot in R
- How to Create and Interpret Histogram in R Studio
Python Programming
- Your First Project in Data Analysis Using Python
- How to Create Boxplot in Python
- How to Create and Interpret Histogram in Python
- How to Calculate Coefficient of Variation in Python
- How to Use ‘With’ Keyword to Open Text File in Python
- Python XOR: Comprehensive Guide to Exclusive OR Operator
So, are you ready to embark on your next data quest?