What is Bitwise AND Operator
Hey there, fellow coder! Welcome to Statssy!
Ready to amp up your programming skills? Today, we’re exploring the Bitwise AND operator, a nifty little tool that’s as practical as it is intriguing. Whether you’re navigating the landscape of binary operations or just want to add another trick to your coding repertoire, Bitwise AND is here to help.
So, what’s the story behind Bitwise AND? In essence, Bitwise AND is a type of operator that returns a True bit (1) only when both of its corresponding bits are True (1). Intrigued? Let’s make it crystal clear with a truth table:
Input A | Input B | A AND B |
---|---|---|
1 | 1 | 1 |
1 | 0 | 0 |
0 | 1 | 0 |
0 | 0 | 0 |
Check out that table! If both inputs (A and B) are 1, Bitwise AND says, “Awesome, I’m a 1 too!” But if either or both are 0, it’s a straight-up “Nope, I’m 0.”
In the binary universe, Bitwise AND is like a strict gatekeeper. It only lets a bit pass if it and its partner are both 1s.
Stick around, and by the end of this article, you’ll be a Bitwise AND guru, wielding this operator like a pro and unlocking new levels of coding mastery!
Eager to learn more? Let’s get started!
Python Bitwise AND Operator
Alright, let’s jump into the next thrilling part of our Bitwise AND exploration!
What is the Bitwise AND Operator?
Think of Bitwise as that meticulous friend who double-checks everything before making a decision. It looks at two bits and says, “You both have to be 1s for me to be a 1. If either of you is a 0, then I’m a 0 too!”
Mathematical Notation vs. Python Notation of bitwise AND operator
Ready for a sprinkle of technicality? In mathematical terms, Bitwise AND is often represented by the symbol ‘∧’. But Python, in its straightforward style, opts for the ampersand ‘&’. So, whenever you see ‘&’ in Python, know that it’s performing the Bitwise AND operation!
Table 1: Comparison of Bitwise AND notations in Mathematics and Python
Notation Type | Symbol |
---|---|
Mathematics | ∧ |
Python | & |
And now, for the grand reveal…
Figure 1: Visual representation of Bitwise AND operation
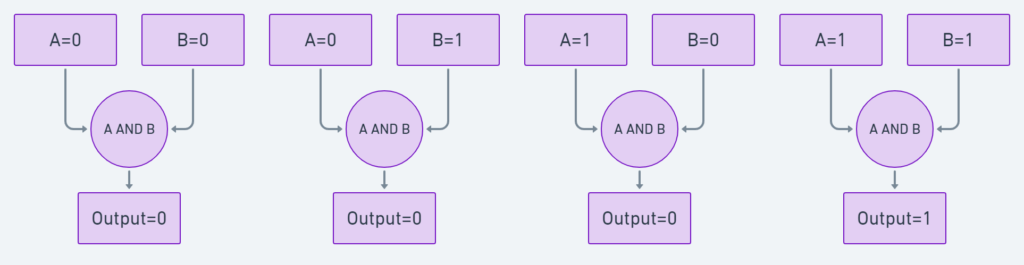
Imagine this diagram showcasing how Bitwise AND scrutinizes its inputs. When both inputs are 1s, Bitwise AND gives a nod of approval (or a 1 in binary). But if either or both inputs are 0s, it’s a firm “Nope, I’m a 0.”
Feeling like a Bitwise AND expert already? Hold tight; we’ve got more to explore! On to more Bitwise AND wonders!
Bitwise AND operator on Integers
Bitwise Operations: A Quick Intro
Before we get into the nitty-gritty, let’s quickly recap what bitwise operations are all about. Imagine each number as a team of bits (1s and 0s). Bitwise operations are like team-building exercises that help these bits interact in specific ways.
How Bitwise AND Compares Bits of Two Integers
Alright, time for some action! Bitwise AND takes two integers and compares their bits one by one, starting from the least significant bit (that’s the one on the far right, just so you know ). If both bits are 1, the result for that position is also a 1. Otherwise, it’s a 0. It’s like a strict teacher who only gives out gold stars when both students get the answer right!
Let’s Dive into an Example!
Ready for some hands-on learning? Let’s say we have two integers, 5 and 3. In binary, 5 is 0101
and 3 is 0011
. Now, let’s perform a Bitwise AND operation:
- 5:
0101
- 3:
0011
- AND:
0001
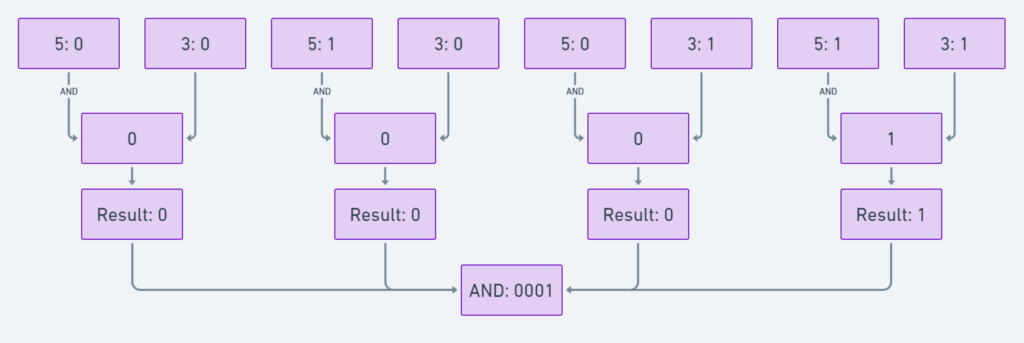
See what happened there? The only time we got a 1 in the result is when both corresponding bits were 1s.
Common AND Operations with Integers
You might be wondering, “When would I ever use this?” Great question! Bitwise AND is often used for tasks like bit masking, which is a fancy way of saying it helps you isolate specific bits in a number. It’s also used in networking to calculate IP addresses and in permissions settings. Basically, it’s a Swiss Army knife in the world of coding!
AND of Booleans
How Bitwise AND Works with Boolean Values
The Bitwise AND operation can also be applied to Boolean values, which are essentially treated as binary digits: True
as 1 and False
as 0. When you perform a Bitwise AND between two Boolean values, the operation will return True
only if both values are True
. If either or both values are False
, the operation will return False
.
Let’s Break It Down with a Truth Table!
To understand this operation more clearly, a truth table can be quite helpful. Here’s how the Bitwise AND operation behaves with Boolean values:
Input A | Input B | A AND B |
---|---|---|
True | True | True |
True | False | False |
False | True | False |
False | False | False |
In this table, you can see that the only scenario where the Bitwise AND operation returns True
is when both Input A and Input B are True
. In all other combinations, the result is False
.
A Quick Example to Seal the Deal (Bitwise AND Operator in Python)
Let’s consider a Python example to demonstrate this operation:
# Using Bitwise AND with Boolean values in Python # When both values are True result1 = True & True print("True & True =", result1) # Output will be True # When one value is True and the other is False result2 = True & False print("True & False =", result2) # Output will be False # When one value is False and the other is True result3 = False & True print("False & True =", result3) # Output will be False # When both values are False result4 = False & False print("False & False =", result4) # Output will be False
In this example, the Bitwise AND operation is performed between different combinations of Boolean values. The operation returns True
only when both Boolean values are True
.
Bit Masking with AND
What is Bit Masking?
Bit masking is a technique used to manipulate specific bits within an integer. It’s like using a stencil to paint: you cover up the parts you don’t want to change, allowing you to focus solely on the bits you’re interested in. This is particularly useful in scenarios like data compression, error detection, and more.
Why Use Bitwise AND for Masking?
Bitwise AND is an effective tool for bit masking because it allows you to isolate specific bits within a number. When you perform a Bitwise AND between a number and a mask, the bits that correspond to the zeros in the mask will be cleared (set to 0), while the bits corresponding to the ones in the mask remain unchanged. This makes Bitwise AND a precise and efficient method for bit manipulation.
Let’s see the technique! : Operator in Python
To better understand, let’s consider an example where we want to isolate the least significant bit (LSB) of an integer. The LSB is the rightmost bit in a binary number.
Here’s how you can do it in Python:
# Python code to demonstrate bit masking with AND def isolate_LSB(number): # Create a mask with only the LSB set to 1 (i.e., 0001 in binary) mask = 1 # Perform Bitwise AND to isolate the LSB isolated_bit = number & mask return isolated_bit # Test the function number = 5 # Binary: 0101 result = isolate_LSB(number) print(f"The least significant bit of {number} is {result}") # Output will be 1
In this example, we define a function `isolate_LSB
` that takes an integer as input. We create a mask with only the LSB set to 1 (which is 1
or 0001
in binary). Then, we perform a Bitwise AND operation between the number and the mask to isolate the LSB.
Bitwise AND Function in Python
What is “operator
” module in Python
The operator
module in Python provides a set of efficient functions corresponding to the intrinsic operators of Python. For example, operator.add(x, y)
is equivalent to the expression x + y
. This module is particularly useful when you need to pass an operator function as an argument to methods like map()
and filter()
.
Using the Bitwise AND Function from the operator
Module
The operator
the module includes a function called and_
that performs a Bitwise AND operation. It takes two arguments and returns their Bitwise AND. Here’s how you can use it:
import operator # Using operator.and_ to perform Bitwise AND result = operator.and_(5, 3) # Equivalent to 5 & 3 print(f"The result of 5 & 3 using operator.and_ is {result}") # Output will be 1
In this example, we import the operator
module and then use its and_
function to perform a Bitwise AND operation between 5 and 3. The result is the same as using the &
operator.
Other Useful Functions in the operator
Module
The operator
module is not just about Bitwise AND; it offers a variety of other useful functions for different operations:
or_
: Performs Bitwise ORxor
: Performs Bitwise XOR (Check out the tutorial here)not_
: Performs Bitwise NOTlshift
: Performs left bitwise shiftrshift
: Performs right bitwise shift
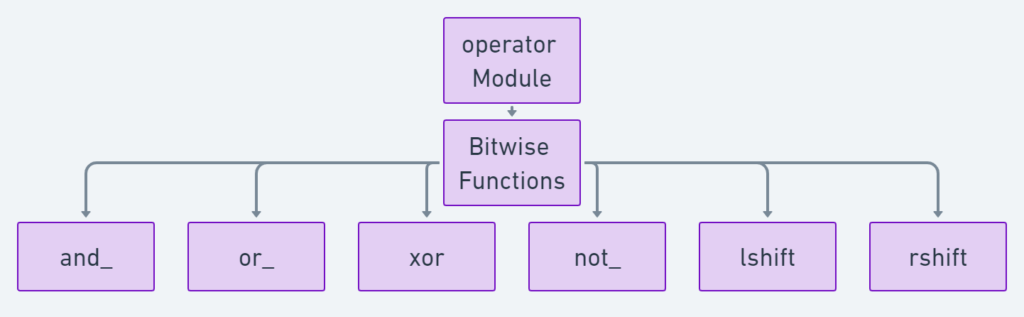
These functions can be used in the same way as and_
, providing a functional approach to these operations.
Conclusion and Further Exploration
Key Takeaways
Understanding Bitwise AND: We’ve learned that the Bitwise AND operation can be applied to both integers and Boolean values, and it plays a crucial role in bit masking.
- Boolean Operations: Bitwise AND returns
True
only when both Boolean values areTrue
. - Bit Masking: The Bitwise AND operation is particularly useful for bit masking, allowing you to manipulate specific bits within an integer.
- Python’s
operator
Module: This module provides a functional approach to Bitwise AND and other bitwise operations, making it easier to pass these operations as arguments to other functions.
Further Exploration
- Other Bitwise Operations: Explore other bitwise operations like OR, XOR, and NOT to get a more rounded understanding of bitwise manipulations.
- Advanced Bit Masking: Dive deeper into bit masking techniques for more complex scenarios, such as setting or clearing specific bits.
- Performance: Investigate the performance implications of using bitwise operations in your code, especially in time-sensitive applications.
Wrapping Up
We’ve covered a lot of ground in this article, from the basics of Bitwise AND to its applications in bit masking and Boolean operations. We also touched on Python’s operator
module as an alternative way to perform these operations. Whether you’re a beginner or an intermediate programmer, understanding bitwise operations can give you a new set of tools for problem-solving in Python.
Frequently Asked Questions (FAQs) on Bitwise AND
1. What is Bit Masking and why is it useful?
Bit masking is a technique used to manipulate specific bits within an integer. By using Bitwise AND with a mask, you can isolate, set, or clear specific bits in a number. This is particularly useful in scenarios like data compression, error detection, and hardware control.
2. Can I use Bitwise AND with data types other than integers and booleans in Python?
In Python, the Bitwise AND operation is primarily designed for integers and booleans. Applying it to other data types like floats or strings will result in a TypeError.
3. How does Bitwise AND differ from Logical AND?
Bitwise AND operates on individual bits of an integer or boolean values, whereas Logical AND (and
in Python) operates at the boolean level, evaluating the truthiness of entire expressions. For example, True and False
returns False
, but True & False
returns 0
.
4. Why would I use the operator
module instead of the &
symbol for Bitwise AND?
The operator
module provides a functional approach to Bitwise AND, which can be useful when you need to pass the operation as an argument to other functions like map()
or filter()
. It offers the same functionality as the &
symbol but in a form that’s easier to pass around in your code.
5. Are there other bitwise operators in Python similar to Bitwise AND?
Yes, Python supports several other bitwise operators, including Bitwise OR (|
), Bitwise XOR (^
), Bitwise NOT (~
), Left Shift (<<
), and Right Shift (>>
). Each of these operators has its own set of use cases and can be equally useful for bit manipulation tasks.